Configuration of Gmail
As of May 30, 2022, Google no longer supports the use of third-party apps or devices which ask you to sign in to your Google Account using only your username and password. Here is the note on Google Policy on less secure apps.
Step 1:
Login to the Dashboard of Google Accounts: https://myaccount.google.com
Step 2:
Ensure that two step verification is switched on:
Step 3:
Click on 'App passwords'. Sign in with email and password. choose the app as mail
Step 4:
Click on GENERATE. Choose the app password thus generated (highlighted in yellow). Follow the instructions on 'how to use it'
Note: Please note that once we enable the 2-Step Verification, the "Less secure app access" automatically stands disabled (as shown in figure below). All the above steps can be done away with if you just enable the "Less secure app access"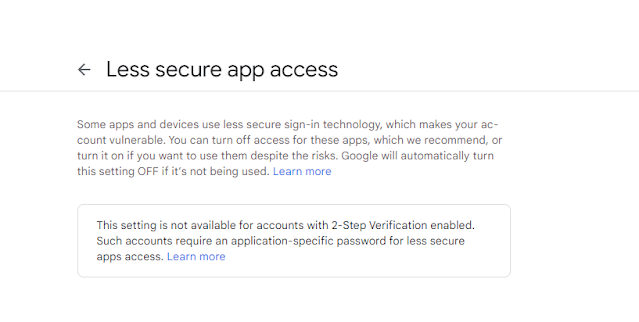
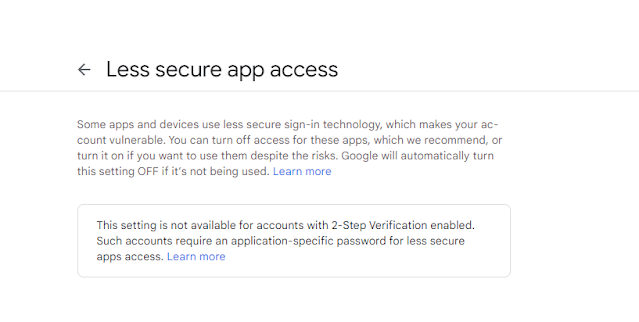
Python Code for email without an attachment
Here is the python code to send a simple email:
# Imports for the program import smtplib # create SMTP session server = smtplib.SMTP('smtp.gmail.com', 587) # smtp address for gmail and port number # start Transport Layer Security(TLS) server.starttls() # Sender email and pass sender_email = "test@gmail.com" sender_password = "testpass" # Authentication server.login(sender_email, sender_password) # Receiver email's receiver_email = ['test1@gmail.com', 'test2@gmail.com'] email_message_string = '''Subject: Mail from Pav This email is a test mail that is sent using Python''' # sending the mail server.sendmail(sender_email, receiver_email, email_message_string) # terminating the session server.quit()
Python code to send HTML email with the email.mime module
We generally use Multipurpose Internet Mail Extensions (MIME) standard to achieve this. MIME is an Internet standard that extends the format of email messages to support text in character sets other than ASCII, as well as attachments of audio, video, images, and application programs
These are the steps that we take:
- Import modules
- Define the HTML document
- Set up the email addresses and password
- Create a MIMEMultipart class, and set up the From, To, Subject fields
- Attach the html doc defined earlier, as a MIMEText html content type to the MIME message
- Convert the email message as string
- Connect to the Gmail SMTP server and send Email
# Imports used in program import smtplib, ssl from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText # Define the HTML document html = ''' <html> <body> <h1>Monthy Report</h1> <p>Hello Shawn, welcome to your monthly report!</p> </body> </html> ''' # Set up the email addresses and password. Please replace below with your email address and password sender_email = "test@gmail.com" sender_password = "testpass" receiver_email = 'test1@gmail.com' # Create a MIMEMultipart class, and set up the From, To, Subject fields email_message = MIMEMultipart() email_message['From'] = sender_email email_message['To'] = receiver_email email_message['Subject'] = 'Email with Monthly report' # Attach the html doc defined earlier, as a MIMEText html content type to the MIME message email_message.attach(MIMEText(html, "html")) # Convert it as a string email_string = email_message.as_string() # Connect to the Gmail SMTP server and Send Email context = ssl.create_default_context() with smtplib.SMTP_SSL("smtp.gmail.com", 465, context=context) as server: server.login(sender_email, sender_password) server.sendmail(sender_email, receiver_email, email_string)
Python Code to send an email with attachment
Let us modify the above code to include an attachment.
# Imports used in program import smtplib, ssl from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText from email.mime.application import MIMEApplication # Define the HTML document html = ''' <html> <body> <h1>Monthly Report</h1> <p>Hello Shawn Johnson, welcome to your monthly report!</p> </body> </html> ''' # Define a function to attach files as MIMEApplication to the email def attach_file_to_email(email_message, filename): # Open the attachment file for reading in binary mode, and make it a MIMEApplication class with open(filename, "rb") as f: file_attachment = MIMEApplication(f.read()) # Add header/name to the attachments file_attachment.add_header( "Content-Disposition", f"attachment; filename= {filename}", ) # Attach the file to the message email_message.attach(file_attachment) # Set up the email addresses and password. Please replace below with your email address and password sender_email = "test@gmail.com" sender_password = "pass" receiver_email = "test2@gmail.com" # Create a MIMEMultipart class, and set up the From, To, Subject fields email_message = MIMEMultipart() email_message['From'] = sender_email email_message['To'] = receiver_email email_message['Subject'] = 'Report email' # Attach the html doc defined earlier, as a MIMEText html content type to the MIME message email_message.attach(MIMEText(html, "html")) # Add attachment attach_file_to_email(email_message, 'TestAttachment.txt') # Convert it as a string email_string = email_message.as_string() # Connect to the Gmail SMTP server and Send Email context = ssl.create_default_context() with smtplib.SMTP_SSL("smtp.gmail.com", 465, context=context) as server: server.login(sender_email, sender_password) server.sendmail(sender_email, receiver_email, email_string)
No comments:
Post a Comment